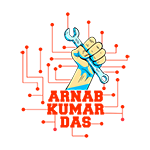 |
Embedded C Firmware Library : Arduino / Atmega328p
1
Register Level Embedded C Hardware Abstraction Library for AVR ATmega48A/PA/88A/PA/168A/PA/328/P or Arduino UNO/NANO/MINI
|
Go to the documentation of this file.
39 #include <avr/interrupt.h>
43 #pragma message ( "avr_usart.h included" )
49 #define USART_SYNCHRONOUS_CLOCK_PRIORITY 0
50 #define USART_MODE_ASYNCHRONOUS (0<<UMSEL00)
51 #define USART_MODE_SYNCHRONOUS (1<<UMSEL00) | USART_SYNCHRONOUS_CLOCK_PRIORITY
52 #define USART_MODE_MASK (1<<UMSEL00)
59 #define USART_PARITY_BIT_NO (0<<UPM00)
60 #define USART_PARITY_BIT_EVEN (1<<UPM01)
61 #define USART_PARITY_BIT_ODD (1<<UPM01) | (1<<UPM00)
62 #define USART_PARITY_BIT_MASK (1<<UPM01) | (1<<UPM00)
69 #define USART_STOP_BIT_ONE (0<<USBS0)
70 #define USART_STOP_BIT_TWO (1<<USBS0)
71 #define USART_STOP_BIT_MASK (1<<USBS0)
78 #define USART_DATA_BIT_FIVE (0<<UCSZ00)
79 #define USART_DATA_BIT_SIX (1<<UCSZ00)
80 #define USART_DATA_BIT_SEVEN (1<<UCSZ01)
81 #define USART_DATA_BIT_EIGHT (1<<UCSZ01) | (1<<UCSZ00)
82 #define USART_DATA_BIT_MASK (1<<UCSZ01) | (1<<UCSZ00)
89 #define USART_RX_COMPLETE_INTERRUPT (1<<RXCIE0)
90 #define USART_TX_COMPLETE_INTERRUPT (1<<TXCIE0)
91 #define USART_DATA_REGISTER_EMPTY_INTERRUPT (1<<UDRIE0)
98 typedef struct USART_ConfigData
112 static volatile uint8_t *USART_TransmitBuffer;
113 static volatile uint8_t USART_TransmitBusyFlag = 0;
114 static volatile uint16_t USART_TransmitBufferIndex = 0;
115 static volatile uint16_t USART_TransmitBufferLength = 0;
117 static volatile uint8_t *USART_ReceiveBuffer;
118 static volatile uint8_t USART_ReceiveBusyFlag = 0;
119 static volatile uint16_t USART_ReceiveBufferIndex = 0;
120 static volatile uint16_t USART_ReceiveBufferLength = 0;
193 for (
int i = 0; i < USART_ReceiveBufferLength; i++)
195 USART_ReceiveBuffer[i] = 0;
198 USART_ReceiveBuffer = 0;
199 USART_ReceiveBusyFlag = 0;
200 USART_ReceiveBufferIndex = 0;
201 USART_ReceiveBufferLength = 0;
211 for (
int i = 0; i < USART_TransmitBufferLength; i++)
213 USART_TransmitBuffer[i] = 0;
216 USART_TransmitBuffer = 0;
217 USART_TransmitBusyFlag = 0;
218 USART_TransmitBufferIndex = 0;
219 USART_TransmitBufferLength = 0;
278 uint16_t USART_BaudPrescaler = 0;
282 USART_BaudPrescaler = (((
F_CPU / (Data.BaudRate * 16UL))) - 1);
286 USART_BaudPrescaler = (((
F_CPU / (Data.BaudRate * 2UL))) - 1);
290 UBRR0H = USART_BaudPrescaler >> 8;
291 UBRR0L = USART_BaudPrescaler;
294 UCSR0C = Data.UsartMode | Data.ParityBit | Data.StopBit | Data.DataBit;
297 UCSR0B = (1<<RXEN0) | (1<<TXEN0);
322 while ((UCSR0A & (1<<RXC0)) == 0);
335 while (USART_ReceiveBusyFlag)
339 USART_ReceiveBuffer = Buffer;
340 USART_ReceiveBufferLength = 1;
341 USART_ReceiveBufferIndex = 0;
342 USART_ReceiveBusyFlag = 1;
355 while (USART_ReceiveBusyFlag)
359 USART_ReceiveBuffer = (uint8_t*)Buffer;
360 USART_ReceiveBufferLength = 2;
361 USART_ReceiveBufferIndex = 0;
362 USART_ReceiveBusyFlag = 1;
376 if (Size == 0)
return ERROR;
378 while (USART_ReceiveBusyFlag)
382 USART_ReceiveBuffer = Buffer;
383 USART_ReceiveBufferLength = Size;
384 USART_ReceiveBufferIndex = 0;
385 USART_ReceiveBusyFlag = 1;
398 while (USART_ReceiveBusyFlag)
402 USART_ReceiveBuffer = (uint8_t*)Character;
403 USART_ReceiveBufferLength = 1;
404 USART_ReceiveBufferIndex = 0;
405 USART_ReceiveBusyFlag = 1;
419 if (Size == 0)
return ERROR;
421 char localbuffer[7] = {0};
422 while (USART_ReceiveBusyFlag)
426 USART_ReceiveBuffer = (uint8_t*)localbuffer;
427 USART_ReceiveBufferLength = Size;
428 USART_ReceiveBufferIndex = 0;
429 USART_ReceiveBusyFlag = 1;
431 while (USART_ReceiveBusyFlag);
445 if (Size == 0)
return ERROR;
447 char localbuffer[12] = {0};
448 while (USART_ReceiveBusyFlag)
452 USART_ReceiveBuffer = (uint8_t*)localbuffer;
453 USART_ReceiveBufferLength = Size;
454 USART_ReceiveBufferIndex = 0;
455 USART_ReceiveBusyFlag = 1;
457 while (USART_ReceiveBusyFlag);
471 if (Size == 0)
return ERROR;
473 while (USART_ReceiveBusyFlag)
477 USART_ReceiveBuffer = (uint8_t*)Buffer;
478 USART_ReceiveBufferLength = Size;
479 USART_ReceiveBufferIndex = 0;
480 USART_ReceiveBusyFlag = 1;
493 USART_ReceiveBusyFlag = 0;
503 while ((UCSR0A & (1<<UDRE0)) == 0);
516 while (USART_TransmitBusyFlag)
520 USART_TransmitBuffer = Buffer;
521 USART_TransmitBufferLength = 1;
522 USART_TransmitBufferIndex = 0;
523 USART_TransmitBusyFlag = 1;
536 while (USART_TransmitBusyFlag)
540 USART_TransmitBuffer = (uint8_t*)Buffer;
541 USART_TransmitBufferLength = 2;
542 USART_TransmitBufferIndex = 0;
543 USART_TransmitBusyFlag = 1;
557 if (Size == 0)
return ERROR;
559 while (USART_TransmitBusyFlag)
563 USART_TransmitBuffer = Buffer;
564 USART_TransmitBufferLength = Size;
565 USART_TransmitBufferIndex = 0;
566 USART_TransmitBusyFlag = 1;
579 uint8_t* data = (uint8_t*)&Character;
591 while (USART_TransmitBusyFlag)
595 static uint8_t localbuffer[7] = {0};
608 while (USART_TransmitBusyFlag)
612 static uint8_t localbuffer[12] = {0};
626 while (USART_TransmitBusyFlag)
630 static uint8_t localbuffer[17] = {0};
643 while (USART_TransmitBusyFlag)
647 const char *p = Buffer;
649 uint16_t Size = p - Buffer;
650 USART_TransmitBuffer = (uint8_t*)Buffer;
651 USART_TransmitBufferLength = Size;
652 USART_TransmitBufferIndex = 0;
653 USART_TransmitBusyFlag = 1;
666 USART_TransmitBusyFlag = 0;
676 if(USART_ReceiveBufferIndex < USART_ReceiveBufferLength)
678 USART_ReceiveBuffer[USART_ReceiveBufferIndex] = UDR0;
679 USART_ReceiveBufferIndex++;
681 if(USART_ReceiveBufferIndex >= USART_ReceiveBufferLength)
683 USART_ReceiveBusyFlag = 0;
695 if(USART_TransmitBufferIndex < USART_TransmitBufferLength)
697 UDR0 = USART_TransmitBuffer[USART_TransmitBufferIndex];
698 USART_TransmitBufferIndex++;
700 if(USART_TransmitBufferIndex >= USART_TransmitBufferLength)
702 USART_TransmitBusyFlag = 0;
uint8_t USART_TransmitBlocking(uint8_t *Buffer)
Public Function to Transmit 1 Byte of Data in Polling.
Definition: avr_usart.h:501
uint8_t USART_TransmitFloat(float Number, uint8_t Precision)
Public Function to Transmit float Data as ASCII.
Definition: avr_usart.h:624
void USART_FlushReceiveBuffer()
Public Function to Flush the Receive Buffer.
Definition: avr_usart.h:191
uint8_t USART_ReceiveLong(long *Number, uint8_t Size)
Public Function to Receive long Data as ASCII.
Definition: avr_usart.h:443
uint8_t USART_ReceiveInt(int *Number, uint8_t Size)
Public Function to Receive int Data as ASCII.
Definition: avr_usart.h:417
void USART_EnableReceiveInterrupt()
Public Function to Enable Receive Interrupt.
Definition: avr_usart.h:247
uint8_t USART_TransmitBytes(uint8_t *Buffer, uint16_t Size)
Public Function to Transmit Multiple Bytes of Data.
Definition: avr_usart.h:555
uint8_t USART_TransmitInt(int Number)
Public Function to Transmit int Data as ASCII.
Definition: avr_usart.h:589
void USART_CancelTransmit()
Public Function to Cancel any Interrupt based Transmission.
Definition: avr_usart.h:663
uint8_t UTILS_IntToString(int Number, char *Buffer)
Public Function to Convert an int Data into a string Data.
Definition: avr_utils.h:93
uint8_t UTILS_LongToString(long Number, char *Buffer)
Public Function to Convert an long Data into a string Data.
Definition: avr_utils.h:123
uint8_t USART_ReceiveBlocking()
Public Function to Receive 1 Byte of Data in Polling.
Definition: avr_usart.h:319
Library for Utility Functions.
uint8_t USART_TransmitString(char *Buffer)
Public Function to Transmit string Data.
Definition: avr_usart.h:641
uint8_t USART_TransmitTwoBytes(uint16_t *Buffer)
Public Function to Transmit 2 Bytes of Data with LSB Byte First.
Definition: avr_usart.h:534
void USART_CancelReceive()
Public Function to Cancel any Interrupt based Reception.
Definition: avr_usart.h:490
uint8_t USART_ReceiveBytes(uint8_t *Buffer, uint16_t Size)
Public Function to Receive Multiple Bytes of Data.
Definition: avr_usart.h:374
int UTILS_StringToInt(char *Buffer)
Public Function to Convert an string Data into a int Data.
Definition: avr_utils.h:181
uint8_t USART_TransmitByte(uint8_t *Buffer)
Public Function to Transmit 1 Byte of Data.
Definition: avr_usart.h:514
int UTILS_StringToLong(char *Buffer)
Public Function to Convert an string Data into a long Data.
Definition: avr_utils.h:208
#define USART_MODE_SYNCHRONOUS
Definition: avr_usart.h:51
#define USART_MODE_ASYNCHRONOUS
Definition: avr_usart.h:50
Library for all common Macro Definition.
void USART_DisableReceiveInterrupt()
Public Function to Disable Receive Interrupt.
Definition: avr_usart.h:257
void USART_EnableTransmitInterrupt()
Public Function to Enable Transmit Interrupt.
Definition: avr_usart.h:227
uint8_t UTILS_FloatToString(float Number, char *Buffer, uint8_t Precision)
Public Function to Convert an float Data into a string Data.
Definition: avr_utils.h:154
#define F_CPU
Definition: avr_macros.h:39
void USART_Init(USART_ConfigData Data)
Public Function to Configure and Initialize USART.
Definition: avr_usart.h:276
uint8_t USART_ReceiveChar(char *Character)
Public Function to Receive char Data.
Definition: avr_usart.h:396
#define USART_DATA_REGISTER_EMPTY_INTERRUPT
Definition: avr_usart.h:91
void USART_FlushTransmitBuffer()
Public Function to Flush the Transmit Buffer.
Definition: avr_usart.h:209
uint8_t USART_ReceiveByte(uint8_t *Buffer)
Public Function to Receive 1 Byte of Data.
Definition: avr_usart.h:333
uint8_t USART_TransmitChar(char Character)
Public Function to Transmit char Data.
Definition: avr_usart.h:577
void USART_DeInit()
Public Function to De-Initialize USART.
Definition: avr_usart.h:308
Definition: avr_usart.h:99
uint8_t USART_ReceiveString(char *Buffer, uint16_t Size)
Public Function to Receive string Data.
Definition: avr_usart.h:469
uint8_t USART_TransmitLong(long Number)
Public Function to Transmit long Data as ASCII.
Definition: avr_usart.h:606
#define USART_RX_COMPLETE_INTERRUPT
Definition: avr_usart.h:89
uint8_t USART_ReceiveTwoBytes(uint16_t *Buffer)
Public Function to Receive 2 Bytes of Data with LSB Byte First.
Definition: avr_usart.h:353
ISR(USART_RX_vect)
Interrupt Service Routine for Reception.
Definition: avr_usart.h:674
void USART_DisableTransmitInterrupt()
Public Function to Disable Transmit Interrupt.
Definition: avr_usart.h:237